Introduction
Imagine creating a text adventure game that writes itself, crafting stories that surprise even you, the developer. Or picture building a digital artist that transforms your words into vivid images. These aren't far-fetched sci-fi scenarios—they're real possibilities when you combine Python programming with generative AI.
Welcome to your Python playground, where we're tossing aside dusty textbooks and diving into learning through creation. If you've ever dozed off during a programming tutorial or felt overwhelmed by endless syntax explanations, this guide is your wake-up call. We're going to learn Python the way your brain actually wants to learn—by building something awesome.
Our vehicle for this learning journey? An AI-powered text adventure generator that will grow in sophistication as your Python skills develop. By the end of this guide, you'll have created a game that leverages generative AI to produce unique storylines, characters, and challenges—all while mastering Python fundamentals without the yawn-inducing theory overload.
Whether you're a complete beginner who thinks "Python" is just a snake, or someone who's tinkered with code but wants to level up for the AI revolution, this guide meets you where you are. We'll cover essential Python concepts like variables, control structures, and functions, but always in the context of our project—no abstract examples about calculating the area of a triangle or counting vowels in a string (unless our adventure hero is trapped in a geometric dungeon or casting vowel-counting spells).
The world of generative AI is exploding with possibilities, and Python is your golden ticket to this revolution. Companies are scrambling to find developers who understand both programming fundamentals and AI concepts. By learning Python with a focus on generative AI applications, you're not just picking up a programming language—you're positioning yourself at the intersection of two of the hottest fields in technology.
So grab your favorite beverage, clear some space on your desk (and in your brain), and prepare to embark on a Python learning adventure that's actually fun. No prior programming experience required—just bring your curiosity and willingness to experiment. Things might break, bugs will appear (the digital kind, not the creepy-crawly ones), and you'll occasionally want to throw your computer out the window—but that's all part of the authentic programming experience we're embracing.
Ready to transform from Python novice to AI game developer? Let's dive in and start coding our adventure!
Setting Up Your Python Environment
Before we dive into creating our AI-powered text adventure, let's get your Python environment ready for action. Think of this as gathering your tools before embarking on an epic quest—except instead of swords and shields, we're collecting code editors and interpreters.
Installing Python
First things first: you need Python on your computer. It's like trying to bake cookies without an oven—technically possible but unnecessarily complicated and likely to end in tears.
- Visit python.org/downloads
- Download the latest version for your operating system (Windows, macOS, or Linux)
- Run the installer, making sure to check the box that says "Add Python to PATH" (this is like telling your computer where to find Python when you call for it)
To verify your installation, open your command prompt or terminal and type:
python --version
If you see something like Python 3.10.x
, you're good to go! If you get an error, try using python3
instead of python
—sometimes they're like twins with different names.
Setting Up a Code Editor
Could you write code in Notepad or TextEdit? Sure, and you could also perform surgery with a butter knife. Technically possible, but why make life harder?
I recommend Visual Studio Code (VS Code) for our adventure:
- Download VS Code from code.visualstudio.com
- Install it on your system
- Open VS Code and install the Python extension (click the Extensions icon on the left sidebar and search for "Python")
This extension gives you superpowers like syntax highlighting (pretty colors that make your code readable), code completion (so you don't have to type everything), and debugging tools (for when things inevitably go wrong).
Creating Your First Python File
Let's create a home for our text adventure project:
- Create a new folder on your computer called
text_adventure_ai
- Open VS Code
- Go to File > Open Folder and select your new folder
- Create a new file by clicking File > New File
- Save it as
game.py
(the.py
extension tells your computer this is a Python file)
Running Your First "Hello, World!" Program
It's tradition to start learning a programming language by making it say "Hello, World!" Who are we to break tradition? Plus, it's a quick way to make sure everything's working.
Type this into your game.py
file:
print("Hello, adventurer! Ready to begin your Python quest?")
To run this masterpiece:
- Open a terminal in VS Code (Terminal > New Terminal)
- Type
python game.py
(orpython3 game.py
if needed)
If you see your greeting message, congratulations! You've officially written and executed Python code. It's not exactly going to win any programming awards yet, but everyone starts somewhere.
Introduction to Virtual Environments
Before we go further, let's talk about virtual environments. Think of them as separate rooms for different projects, so they don't mess with each other's stuff.
To create a virtual environment:
# Navigate to your project folder in the terminal cd path/to/text_adventure_ai # Create a virtual environment python -m venv venv # Activate it (the command differs by operating system) # On Windows: venv\Scripts\activate # On macOS/Linux: source venv/bin/activate
When activated, you'll see (venv)
at the beginning of your terminal prompt, like a little badge saying "I'm being responsible with my dependencies!"
Now let's install our first package—we'll need it later for our AI integration:
pip install requests
The requests
library will help us communicate with AI services. Think of it as our project's translator for talking to the outside world.
With our environment set up, we're ready to start building our text adventure generator. In the next section, we'll dive into Python fundamentals while laying the groundwork for our game. Onward to glory!
Python Fundamentals Through Our Project
Now that our coding environment is ready, let's start building our text adventure while learning Python fundamentals. Instead of learning concepts in isolation, we'll see how they work together in our game.
Variables and Data Types: Storing Game Information
In Python, variables are like labeled containers that hold information. Our game needs to keep track of many things: the player's name, their location, inventory items, and more.
Let's start by creating some basic variables for our game:
# game.py # String variables - for text player_name = "Adventurer" current_location = "The Mysterious Forest" game_title = "The AI Chronicles: A Text Adventure" # Integer variables - for numbers without decimal points player_health = 100 gold_coins = 50 days_survived = 1 # Float variables - for numbers with decimal points magic_power = 75.5 enemy_difficulty = 3.7 # Boolean variables - for yes/no, true/false conditions has_magic_sword = False has_met_wizard = True game_active = True # Printing game information using our variables print(f"Welcome to {game_title}!") print(f"You are {player_name}, currently in {current_location}.") print(f"Health: {player_health}, Gold: {gold_coins}, Magic: {magic_power}") print(f"Days survived: {days_survived}") print(f"Magic sword in inventory: {has_magic_sword}")
Notice the f
before the quotation marks in the print statements? That creates an f-string (formatted string), allowing us to embed variables directly in our text. It's like having a fill-in-the-blank template where Python automatically inserts the values.
Run this code, and you'll see a summary of your character's starting state. Not exactly Skyrim, but we're getting there!
Control Structures: Making Game Decisions
If-Else Statements: Conditional Logic
In games, different things happen based on conditions. If you have a key, you can open the door. If your health is low, you need a potion. Let's implement some game logic:
# Adding decision logic to our game # Check player health and show appropriate message if player_health < 20: print("WARNING: Health critically low! Find healing soon!") elif player_health < 50: print("You're wounded. Consider finding health potions.") else: print("Health status: Good") # Check if player has required items for a quest if has_magic_sword and player_health > 75: print("You are ready to face the dragon!") elif has_magic_sword: print("You have the magic sword, but should heal before facing the dragon.") elif player_health > 75: print("You're healthy, but need to find the magic sword before facing the dragon.") else: print("You need both the magic sword and better health before facing the dragon.")
The if
, elif
(else if), and else
statements create decision trees in our code. The program checks each condition in order and executes the first block where the condition is True
.
For Loops: Iteration
Loops help us repeat actions without copying and pasting code. Let's use a for
loop to display the player's inventory:
# Player's inventory as a list inventory = ["Health Potion", "Map", "Torch", "Dagger", "Mysterious Amulet"] print("\nINVENTORY:") for item in inventory: print(f"- {item}") # Counting items with a different loop style print(f"\nYou have {len(inventory)} items in your inventory.") for i in range(len(inventory)): print(f"Item {i+1}: {inventory[i]}")
The first loop iterates directly through the items, while the second uses range()
to generate indices (0, 1, 2, etc.) and then accesses each item by its position. Both accomplish similar goals but in different ways.
While Loops: Continuous Actions
While loops keep executing as long as a condition remains true. They're perfect for the main game loop:
# A simple game loop turns_remaining = 3 while turns_remaining > 0 and game_active: print(f"\n--- Turn {4-turns_remaining} ---") print(f"You are in {current_location}. What will you do?") # Normally we'd get player input here, but for now we'll simulate it print("Simulating player action: 'explore'") # Update game state turns_remaining -= 1 if turns_remaining == 1: current_location = "The Enchanted Cave" print("You discovered an Enchanted Cave!") # Check if game should end if turns_remaining == 0: print("\nEnd of demo. Thanks for playing!") game_active = False
This creates a simple turn-based system where the game continues until we run out of turns or set game_active
to False
.
Functions: Creating Reusable Game Components
Functions are reusable blocks of code that perform specific tasks. They help organize our code and prevent repetition. Let's create some game functions:
# Functions for our text adventure def display_stats(): """Display the player's current statistics.""" print(f"\n--- {player_name}'s Stats ---") print(f"Health: {player_health}") print(f"Gold: {gold_coins}") print(f"Magic Power: {magic_power}") print(f"Days Survived: {days_survived}") def describe_location(location): """Provide a description of the given location.""" descriptions = { "The Mysterious Forest": "A dense forest with towering trees. Strange sounds echo in the distance.", "The Enchanted Cave": "A glittering cave with crystals that emit soft light. The air feels magical.", "The Ancient Temple": "Crumbling stone walls covered in mysterious symbols. An aura of power remains." } if location in descriptions: return descriptions[location] else: return "An unremarkable area with nothing of interest." def encounter_enemy(enemy_type): """Simulate a basic enemy encounter.""" enemy_health = {"Goblin": 30, "Wolf": 40, "Troll": 80}.get(enemy_type, 50) print(f"\nYou encountered a {enemy_type}!") print(f"The {enemy_type} has {enemy_health} health.") print("Combat simulation: You swing your weapon...") # Simulate simple combat outcome import random attack_power = random.randint(20, 50) print(f"You dealt {attack_power} damage!") if attack_power >= enemy_health: print(f"You defeated the {enemy_type}!") return True else: print(f"The {enemy_type} survived with {enemy_health - attack_power} health and ran away!") return False # Using our functions display_stats() print("\n" + describe_location(current_location)) victory = encounter_enemy("Goblin") if victory: gold_coins += 15 print(f"You found 15 gold coins! Total gold: {gold_coins}")
Notice the triple-quoted strings after each function definition? Those are docstrings—documentation that explains what the function does. They're good practice and help other developers (or future you) understand your code.
Data Structures: Organizing Game Information
Lists: Ordered Collections
We've already seen a list with our inventory. Lists are ordered collections that can hold any type of data:
# More examples of lists in our game # List of possible locations locations = ["The Mysterious Forest", "The Enchanted Cave", "The Ancient Temple", "The Dragon's Lair", "The Forgotten Village"] # List of enemy types with different properties (nested lists) enemies = [ ["Goblin", 30, 10], # [name, health, damage] ["Wolf", 40, 15], ["Troll", 80, 20], ["Dragon", 200, 40] ] # Accessing list elements next_location = locations[2] # "The Ancient Temple" (remember, indexing starts at 0) print(f"\nYour next destination: {next_location}") # Modifying lists print("\nEnemies in this region:") for enemy in enemies: print(f"- {enemy[0]}: {enemy[1]} HP, {enemy[2]} damage") # Adding new items inventory.append("Gold Key") print(f"\nYou found a Gold Key! Updated inventory: {inventory}") # Removing items used_item = inventory.pop(0) # Removes and returns "Health Potion" print(f"You used a {used_item}. Remaining inventory: {inventory}")
Dictionaries: Key-Value Pairs
Dictionaries store data as key-value pairs, perfect for mapping names to descriptions or properties:
# Dictionaries for game data # Location details location_data = { "The Mysterious Forest": { "description": "A dense forest with towering trees. Strange sounds echo in the distance.", "danger_level": 3, "available_exits": ["The Enchanted Cave", "The Forgotten Village"], "items": ["Mushroom", "Wooden Shield"] }, "The Enchanted Cave": { "description": "A glittering cave with crystals that emit soft light. The air feels magical.", "danger_level": 5, "available_exits": ["The Mysterious Forest", "The Ancient Temple"], "items": ["Magic Crystal", "Silver Ring"] } } # Character abilities player_abilities = { "Fireball": {"damage": 40, "mana_cost": 15}, "Heal": {"health_restored": 30, "mana_cost": 20}, "Shield": {"defense_bonus": 25, "duration": 3, "mana_cost": 10} } # Using dictionaries current_place = "The Enchanted Cave" place_info = location_data[current_place] print(f"\n--- {current_place} ---") print(place_info["description"]) print(f"Danger Level: {place_info['danger_level']}/10") print("Exits available:", ", ".join(place_info["available_exits"])) print("Items found here:", ", ".join(place_info["items"])) # Player using an ability chosen_ability = "Fireball" if chosen_ability in player_abilities: ability_info = player_abilities[chosen_ability] print(f"\nYou cast {chosen_ability}!") print(f"It deals {ability_info['damage']} damage and costs {ability_info['mana_cost']} mana.") magic_power -= ability_info["mana_cost"] print(f"Remaining magic power: {magic_power}")
Dictionaries use curly braces {}
and store data as key: value
pairs. They're incredibly useful for complex data that needs to be accessed by name rather than position.
Error Handling: Preventing Game Crashes
Even the best-written games can encounter unexpected situations. Error handling helps our game gracefully manage problems:
# Error handling examples def use_item(item_name): """Attempt to use an item from inventory.""" try: item_index = inventory.index(item_name) used_item = inventory.pop(item_index) print(f"You used the {used_item}.") # Item effects would go here if used_item == "Health Potion": return "Restored 30 health!" elif used_item == "Magic Crystal": return "Gained 25 magic power!" else: return "Nothing happened." except ValueError: return f"You don't have a {item_name} in your inventory!" except Exception as e: return f"Error: {str(e)}" # Testing our error handling print("\n" + use_item("Magic Crystal")) # Should work if we have it print(use_item("Health Potion")) # We already used this, so should show error print(use_item("Legendary Sword")) # We don't have this item # Safe input parsing def get_player_choice(prompt, options): """Safely get a valid choice from the player.""" print(f"\n{prompt}") for i, option in enumerate(options, 1): print(f"{i}. {option}") while True: try: choice = int(input("\nEnter your choice (number): ")) if 1 <= choice <= len(options): return options[choice-1] else: print(f"Please enter a number between 1 and {len(options)}.") except ValueError: print("Please enter a valid number.") # We won't call this function yet since it requires user input # But it shows how we'd handle player choices safely
The try
/except
blocks let us attempt operations that might fail and handle any errors gracefully. This prevents our game from crashing when something unexpected happens.
Building Our Text Adventure Generator
Now that we've covered the fundamentals, let's start building our AI-powered text adventure generator. We'll begin with a basic structure and gradually enhance it with AI capabilities.
Step 1: Setting Up the Basic Game Structure
Let's create a new file called adventure_game.py
with our core game structure:
# adventure_game.py import random import time class TextAdventure: def __init__(self, player_name): self.player_name = player_name self.health = 100 self.inventory = ["Map", "Torch", "Water Flask"] self.current_location = "Starting Point" self.game_active = True # Basic story elements self.locations = ["Forest", "Cave", "Mountain", "Castle", "Village"] self.characters = ["Wizard", "Merchant", "Knight", "Mysterious Stranger"] self.items = ["Sword", "Shield", "Potion", "Ancient Book", "Magic Amulet"] def display_status(self): """Show the player's current status.""" print(f"\n--- {self.player_name}'s Adventure ---") print(f"Location: {self.current_location}") print(f"Health: {self.health}") print(f"Inventory: {', '.join(self.inventory)}") def generate_description(self, location): """Generate a basic description for a location.""" descriptions = { "Forest": "A dense forest with towering trees. Sunlight barely penetrates the canopy.", "Cave": "A dark, damp cave with echoing sounds of water dripping.", "Mountain": "A rugged mountain path with breathtaking views of the valley below.", "Castle": "An imposing stone castle with high towers and a deep moat.", "Village": "A quaint village with thatched-roof cottages and friendly inhabitants.", "Starting Point": "A crossroads surrounded by rolling hills. Your journey begins here." } return descriptions.get(location, "An unremarkable area with nothing of interest.") def generate_encounter(self): """Generate a random encounter.""" encounter_types = ["character", "item", "enemy", "puzzle"] encounter_type = random.choice(encounter_types) if encounter_type == "character": character = random.choice(self.characters) return f"You meet a {character} who offers you advice about the dangers ahead." elif encounter_type == "item": item = random.choice(self.items) if item not in self.inventory: self.inventory.append(item) return f"You found a {item}! It has been added to your inventory." else: return f"You spot a {item}, but you already have one." elif encounter_type == "enemy": enemies = ["Goblin", "Wolf", "Bandit", "Skeleton"] enemy = random.choice(enemies) damage = random.randint(5, 20) self.health -= damage return f"A {enemy} attacks you! You lose {damage} health points in the battle." else: # puzzle return "You encounter a strange puzzle. After some thought, you manage to solve it." def move_to_new_location(self): """Move to a new location.""" new_location = random.choice(self.locations) while new_location == self.current_location: new_location = random.choice(self.locations) self.current_location = new_location return f"You travel to the {new_location}.\n{self.generate_description(new_location)}" def get_player_choice(self): """Get the player's next action choice.""" print("\nWhat would you like to do?") print("1. Explore current location") print("2. Move to a new location") print("3. Check status") print("4. Quit game") while True: try: choice = int(input("\nEnter your choice (1-4): ")) if 1 <= choice <= 4: return choice else: print("Please enter a number between 1 and 4.") except ValueError: print("Please enter a valid number.") def play_turn(self): """Play a single turn of the game.""" choice = self.get_player_choice() if choice == 1: # Explore print("\n" + self.generate_encounter()) elif choice == 2: # Move print("\n" + self.move_to_new_location()) elif choice == 3: # Check status self.display_status() elif choice == 4: # Quit self.game_active = False print("\nThanks for playing!") # Check if player has lost if self.health <= 0: print("\nYour health has dropped to zero. Game over!") self.game_active = False def play_game(self): """Main game loop.""" print(f"\nWelcome, {self.player_name}, to your text adventure!") print(self.generate_description(self.current_location)) while self.game_active: self.play_turn() time.sleep(1) # Small pause between turns # Run the game if this file is executed directly if __name__ == "__main__": player_name = input("Enter your character's name: ") game = TextAdventure(player_name) game.play_game()
This creates a basic text adventure game with:
- A player with health and inventory
- Multiple locations with descriptions
- Random encounters with characters, items, and enemies
- A turn-based gameplay system
- Player choices for exploring, moving, and checking status
Try running this game and play through a few turns to see how it works!
Step 2: Creating the Story Generation System
Now, let's enhance our game with a more sophisticated story generation system. We'll create a new file called story_generator.py
:
# story_generator.py import random class StoryGenerator: def __init__(self): # Story elements self.themes = ["Redemption", "Discovery", "Revenge", "Survival", "Mystery"] self.plot_points = [ "A mysterious stranger offers help", "An ancient prophecy is revealed", "A betrayal by a trusted ally", "A magical artifact is discovered", "A long-lost relative returns", "A kingdom in peril needs saving", "A curse must be broken" ] self.challenges = [ "Solve an ancient riddle", "Defeat a powerful enemy", "Navigate a treacherous landscape", "Win the trust of suspicious allies", "Find a hidden object of power", "Escape from a dangerous trap", "Survive against overwhelming odds" ] def generate_story_hook(self): """Generate an initial story hook.""" theme = random.choice(self.themes) plot = random.choice(self.plot_points) hooks = [ f"Your journey of {theme.lower()} begins when {plot.lower()}.", f"In a world where {theme.lower()} is rare, {plot.lower()}.", f"You never sought {theme.lower()}, but {plot.lower()}, changing everything.", f"The path to {theme.lower()} is dangerous, and now {plot.lower()}." ] return random.choice(hooks) def generate_location_description(self, location_name): """Generate a rich description for a location.""" time_of_day = random.choice(["morning", "afternoon", "evening", "night"]) weather = random.choice(["clear", "rainy", "foggy", "stormy", "windy"]) atmosphere = random.choice(["peaceful", "tense", "mysterious", "dangerous", "magical"]) descriptions = { "Forest": [ f"A {atmosphere} forest stretches before you. The {time_of_day} {weather} creates shadows that dance between ancient trees.", f"Towering trees surround you in this {atmosphere} forest. It's {time_of_day}, and the {weather} weather makes the leaves rustle.", f"The forest is {atmosphere} in the {time_of_day} light. {weather.capitalize()} conditions make the path ahead unclear." ], "Cave": [ f"The {atmosphere} cave opening looms before you. {weather.capitalize()} conditions outside contrast with the stillness within.", f"Stalactites hang from the ceiling of this {atmosphere} cave. The {time_of_day} light barely penetrates the entrance.", f"A {atmosphere} darkness fills this cave. The {weather} sounds from outside echo faintly through the tunnels." ], "Mountain": [ f"The {atmosphere} mountain path winds upward. The {time_of_day} {weather} conditions affect visibility.", f"From this {atmosphere} mountain vantage point, you can see for miles. The {time_of_day} {weather} creates a dramatic landscape.", f"The mountain feels {atmosphere} as you climb higher. The {time_of_day} brings {weather} conditions that challenge your journey." ], "Castle": [ f"The {atmosphere} castle stands imposingly against the {time_of_day} sky. {weather.capitalize()} conditions surround its ancient walls.", f"Flags flutter in the {weather} breeze atop this {atmosphere} castle. The {time_of_day} light casts long shadows across the courtyard.", f"The castle's {atmosphere} halls echo with history. Outside, the {time_of_day} brings {weather} conditions." ], "Village": [ f"The village has a {atmosphere} atmosphere in the {time_of_day}. Villagers adapt their routines to the {weather} conditions.", f"Smoke rises from chimneys in this {atmosphere} village. The {time_of_day} {weather} weather influences the bustling activity.", f"The {atmosphere} village square is the center of activity. {weather.capitalize()} conditions in the {time_of_day} affect the mood." ], "Starting Point": [ f"The crossroads has a {atmosphere} feel to it. The {time_of_day} {weather} marks the beginning of your journey.", f"Your adventure begins at this {atmosphere} crossroads. The {time_of_day} brings {weather} conditions as you consider your path.", f"Multiple paths extend from this {atmosphere} starting point. The {time_of_day} {weather} seems symbolic of choices ahead." ] } location_descriptions = descriptions.get(location_name, [f"This {atmosphere} area is unremarkable. The {time_of_day} brings {weather} conditions."]) return random.choice(location_descriptions) def generate_character_encounter(self, character_type): """Generate a character encounter description.""" motivations = ["seeking help", "offering assistance", "sharing information", "requesting a favor", "warning of danger"] appearances = ["well-dressed", "disheveled", "mysterious", "friendly", "suspicious", "wounded", "elderly", "youthful"] motivation = random.choice(motivations) appearance = random.choice(appearances) return f"You encounter a {appearance} {character_type} who is {motivation}. Their eyes suggest they have seen much in their travels." def generate_challenge(self): """Generate a challenge for the player.""" challenge = random.choice(self.challenges) difficulty = random.choice(["seemingly impossible", "difficult", "challenging but doable", "straightforward if you're clever"]) reward = random.choice(["valuable treasure", "powerful artifact", "crucial information", "new ally", "enhanced ability"]) return f"You must {challenge.lower()}, a {difficulty} task. Success will bring {reward}." def generate_plot_twist(self): """Generate a plot twist.""" twists = [ "The villain is actually trying to save the world in their own misguided way.", "Your trusted mentor has been deceiving you from the beginning.", "The magical artifact you seek is actually a trap.", "The true enemy is not who you thought it was.", "Your character discovers they have a special lineage or power.", "A seemingly unimportant character turns out to be crucial to your quest.", "The simple quest reveals itself to be part of a much larger conflict." ] return random.choice(twists) # Example usage if __name__ == "__main__": generator = StoryGenerator() print("Story Hook:", generator.generate_story_hook()) print("\nForest Description:", generator.generate_location_description("Forest")) print("\nCharacter Encounter:", generator.generate_character_encounter("Wizard")) print("\nChallenge:", generator.generate_challenge()) print("\nPlot Twist:", generator.generate_plot_twist())
This creates a more sophisticated story generation system with:
- Varied themes and plot points
- Rich location descriptions that consider time of day, weather, and atmosphere
- Detailed character encounters
- Challenging quests with rewards
- Unexpected plot twists
Step 3: Integrating the Story Generator with Our Game
Now, let's update our main game file to use the story generator:
# enhanced_adventure_game.py import random import time from story_generator import StoryGenerator class EnhancedTextAdventure: def __init__(self, player_name): self.player_name = player_name self.health = 100 self.magic = 50 self.inventory = ["Map", "Torch", "Water Flask"] self.current_location = "Starting Point" self.game_active = True self.turn_count = 0 self.story_progress = 0 # Story elements self.locations = ["Forest", "Cave", "Mountain", "Castle", "Village"] self.characters = ["Wizard", "Merchant", "Knight", "Mysterious Stranger", "Elder", "Child", "Guard"] self.items = ["Sword", "Shield", "Potion", "Ancient Book", "Magic Amulet", "Crystal", "Key"] # Initialize story generator self.story_gen = StoryGenerator() # Generate main quest self.main_quest = self.story_gen.generate_story_hook() self.plot_twist_revealed = False self.plot_twist = self.story_gen.generate_plot_twist() def display_status(self): """Show the player's current status.""" print(f"\n--- {self.player_name}'s Adventure ---") print(f"Location: {self.current_location}") print(f"Health: {self.health}") print(f"Magic: {self.magic}") print(f"Inventory: {', '.join(self.inventory)}") print(f"Quest: {self.main_quest}") def generate_encounter(self): """Generate a random encounter.""" encounter_types = ["character", "item", "enemy", "puzzle", "rest"] encounter_type = random.choice(encounter_types) if encounter_type == "character": character = random.choice(self.characters) return self.story_gen.generate_character_encounter(character) elif encounter_type == "item": item = random.choice(self.items) if item not in self.inventory: self.inventory.append(item) return f"You found a {item}! It has been added to your inventory." else: return f"You spot a {item}, but you already have one." elif encounter_type == "enemy": enemies = ["Goblin", "Wolf", "Bandit", "Skeleton", "Troll", "Dark Mage"] enemy = random.choice(enemies) damage = random.randint(5, 20) self.health -= damage # Combat narrative combat_narratives = [ f"A {enemy} ambushes you from the shadows! After a fierce struggle, you defeat it but suffer {damage} damage.", f"You hear a growl and turn to face a {enemy}. The battle is tough, and you take {damage} damage before emerging victorious.", f"A {enemy} blocks your path, weapons drawn. The ensuing fight leaves you with {damage} damage, but the {enemy} lies defeated." ] return random.choice(combat_narratives) elif encounter_type == "puzzle": return self.story_gen.generate_challenge() else: # rest health_gain = random.randint(5, 15) magic_gain = random.randint(5, 15) self.health = min(100, self.health + health_gain) self.magic = min(100, self.magic + magic_gain) rest_narratives = [ f"You find a peaceful clearing and take time to rest. You recover {health_gain} health and {magic_gain} magic.", f"A friendly hermit offers you shelter for a few hours. You recover {health_gain} health and {magic_gain} magic.", f"You discover a healing spring and take time to recuperate. You recover {health_gain} health and {magic_gain} magic." ] return random.choice(rest_narratives) def move_to_new_location(self): """Move to a new location.""" available_locations = [loc for loc in self.locations if loc != self.current_location] new_location = random.choice(available_locations) self.current_location = new_location return self.story_gen.generate_location_description(new_location) def get_player_choice(self): """Get the player's next action choice.""" print("\nWhat would you like to do?") print("1. Explore current location") print("2. Move to a new location") print("3. Check status") print("4. Quit game") while True: try: choice = int(input("\nEnter your choice (1-4): ")) if 1 <= choice <= 4: return choice else: print("Please enter a number between 1 and 4.") except ValueError: print("Please enter a valid number.") def advance_story(self): """Advance the main story based on turn count.""" self.turn_count += 1 self.story_progress = min(100, self.story_progress + random.randint(5, 10)) # Reveal plot twist around the middle of the game if self.story_progress >= 50 and not self.plot_twist_revealed: print("\n--- PLOT TWIST ---") print(self.plot_twist) self.plot_twist_revealed = True return True # Random story events if random.random() < 0.2: # 20% chance each turn story_events = [ "You find a torn page from an ancient book that hints at your destiny.", "A dream visits you in the night, showing glimpses of what's to come.", "Locals whisper about recent strange events that seem connected to your quest.", "You notice symbols that keep appearing, suggesting a pattern to your journey." ] print("\n--- STORY EVENT ---") print(random.choice(story_events)) return True return False def play_turn(self): """Play a single turn of the game.""" choice = self.get_player_choice() if choice == 1: # Explore print("\n" + self.generate_encounter()) elif choice == 2: # Move print("\n" + self.move_to_new_location()) elif choice == 3: # Check status self.display_status() elif choice == 4: # Quit self.game_active = False print("\nThanks for playing!") return # Advance story self.advance_story() # Check if player has lost if self.health <= 0: print("\nYour health has dropped to zero. Game over!") self.game_active = False # Check if story is complete if self.story_progress >= 100: print("\n--- QUEST COMPLETE ---") print("You have completed your adventure successfully!") print(f"Final status: Health {self.health}, Magic {self.magic}") print(f"Items collected: {', '.join(self.inventory)}") self.game_active = False def play_game(self): """Main game loop.""" print(f"\nWelcome, {self.player_name}, to your text adventure!") print("\n--- YOUR QUEST ---") print(self.main_quest) print("\n--- STARTING LOCATION ---") print(self.story_gen.generate_location_description(self.current_location)) while self.game_active: self.play_turn() time.sleep(1) # Small pause between turns # Run the game if this file is executed directly if __name__ == "__main__": player_name = input("Enter your character's name: ") game = EnhancedTextAdventure(player_name) game.play_game()
This enhanced version integrates our story generator to create a more immersive experience with:
- A main quest with plot twists
- Rich location descriptions
- Varied character encounters
- Story progression that unfolds over time
- Random story events that add depth to the narrative
Step 4: Adding Basic AI Elements with Simple Rules
Before we connect to external AI services, let's add some basic AI-like behavior using rules and randomization. Create a new file called simple_ai.py
:
# simple_ai.py import random import re class SimpleAI: def __init__(self): self.player_history = [] self.world_state = { "danger_level": 3, "magic_prevalence": 5, "npc_friendliness": 7, "weather_severity": 4, "time_of_day": "day" } # Response templates self.combat_templates = [ "The {enemy} attacks with {attack}! You defend with {defense} and counter-attack.", "You spot the {enemy} before it sees you, giving you the advantage. Your {defense} prepares you for its {attack}.", "The {enemy} lunges with a powerful {attack}! Your {defense} is tested to its limits." ] self.npc_templates = [ "The {npc} approaches you cautiously. \"{greeting}\" they say, {demeanor}.", "You find the {npc} {activity}. They look at you and say, \"{greeting}\"", "A {npc} emerges from {direction}. \"{greeting}\" they call out, {demeanor}." ] # Word banks self.enemy_attacks = ["slashing claws", "poisoned blade", "fiery breath", "dark magic", "crushing strength"] self.player_defenses = ["quick reflexes", "magical shield", "sturdy armor", "tactical dodge", "counter-spell"] self.npc_greetings = ["Hail, traveler!", "Well met, stranger.", "You're not from around here, are you?", "Thank the gods you're here!"] self.npc_demeanors = ["smiling warmly", "eyeing you suspiciously", "clearly relieved to see another person", "hiding something behind their back"] self.npc_activities = ["tending a small garden", "repairing a broken tool", "reading an ancient text", "preparing a meal", "keeping watch"] self.directions = ["the shadows", "a nearby building", "the forest path", "behind a large rock", "a hidden doorway"] def record_player_action(self, action): """Record player actions to inform future responses.""" self.player_history.append(action) # Analyze action to update world state if "attack" in action.lower() or "fight" in action.lower(): self.world_state["danger_level"] = min(10, self.world_state["danger_level"] + 1) if "magic" in action.lower() or "spell" in action.lower(): self.world_state["magic_prevalence"] = min(10, self.world_state["magic_prevalence"] + 1) if "help" in action.lower() or "assist" in action.lower(): self.world_state["npc_friendliness"] = min(10, self.world_state["npc_friendliness"] + 1) # Time progression if len(self.player_history) % 5 == 0: # Every 5 actions if self.world_state["time_of_day"] == "day": self.world_state["time_of_day"] = "dusk" elif self.world_state["time_of_day"] == "dusk": self.world_state["time_of_day"] = "night" elif self.world_state["time_of_day"] == "night": self.world_state["time_of_day"] = "dawn" else: self.world_state["time_of_day"] = "day" def generate_combat_narrative(self, enemy_type): """Generate a combat narrative based on the enemy type and world state.""" template = random.choice(self.combat_templates) # Select details based on enemy and world state attack = random.choice(self.enemy_attacks) defense = random.choice(self.player_defenses) # Adjust difficulty based on world state difficulty_adj = ["desperate", "challenging", "manageable", "straightforward"][min(3, self.world_state["danger_level"] // 3)] # Generate the narrative narrative = template.format(enemy=enemy_type, attack=attack, defense=defense) narrative += f" The battle is {difficulty_adj}." # Add time of day and weather effects if self.world_state["time_of_day"] == "night": narrative += " The darkness makes the fight even more treacherous." if self.world_state["weather_severity"] > 7: narrative += " The severe weather conditions complicate your movements." return narrative def generate_npc_interaction(self, npc_type): """Generate an NPC interaction based on the NPC type and world state.""" template = random.choice(self.npc_templates) # Select details greeting = random.choice(self.npc_greetings) demeanor = random.choice(self.npc_demeanors) activity = random.choice(self.npc_activities) direction = random.choice(self.directions) # Adjust friendliness based on world state if self.world_state["npc_friendliness"] > 7: demeanor = "smiling warmly" elif self.world_state["npc_friendliness"] < 4: demeanor = "eyeing you suspiciously" # Generate the interaction interaction = template.format(npc=npc_type, greeting=greeting, demeanor=demeanor, activity=activity, direction=direction) # Add time-specific details if self.world_state["time_of_day"] == "night": interaction += " They carry a flickering lantern that casts long shadows." elif self.world_state["time_of_day"] == "dusk": interaction += " The fading light gives their features an otherworldly quality." return interaction def generate_weather_description(self): """Generate a weather description based on world state.""" severity = self.world_state["weather_severity"] time = self.world_state["time_of_day"] if severity <= 3: if time == "day": return "The sky is clear, with gentle sunlight warming your path." elif time == "night": return "The night is clear, with stars twinkling brightly overhead." else: return "The sky is clear, with a gentle breeze in the air." elif severity <= 6: if time == "day": return "Scattered clouds drift across the sky, occasionally blocking the sun." elif time == "night": return "Clouds obscure parts of the night sky, with moonlight filtering through gaps." else: return "The weather is mild, with a mix of clouds and clear patches." else: if time == "day": return "Dark storm clouds loom overhead, threatening rain at any moment." elif time == "night": return "The night is turbulent, with howling winds and threatening clouds." else: return "The weather is harsh, making travel difficult and visibility poor." def respond_to_input(self, player_input): """Generate a response based on player input.""" self.record_player_action(player_input) # Look for keywords in player input if re.search(r'\b(attack|fight|battle)\b', player_input, re.I): enemies = ["goblin", "wolf", "bandit", "troll", "dark mage", "skeleton"] return self.generate_combat_narrative(random.choice(enemies)) elif re.search(r'\b(talk|speak|ask|greet)\b', player_input, re.I): npcs = ["villager", "merchant", "guard", "child", "elder", "traveler"] return self.generate_npc_interaction(random.choice(npcs)) elif re.search(r'\b(look|examine|observe|see)\b', player_input, re.I): return self.generate_weather_description() + " " + self.describe_surroundings() else: # Generic responses for other inputs responses = [ "You proceed cautiously, alert for any signs of danger.", "The path ahead seems to hold both promise and peril.", "You take a moment to gather your thoughts before continuing.", "Your instincts tell you there's more to this situation than meets the eye." ] return random.choice(responses) def describe_surroundings(self): """Generate a description of the surroundings based on world state.""" time = self.world_state["time_of_day"] danger = self.world_state["danger_level"] magic = self.world_state["magic_prevalence"] descriptions = [] # Time-based descriptions if time == "day": descriptions.append("Sunlight filters through the canopy above.") elif time == "night": descriptions.append("The darkness is punctuated by mysterious sounds.") elif time == "dusk": descriptions.append("Long shadows stretch across the ground as the light fades.") else: # dawn descriptions.append("The first light of day begins to illuminate your surroundings.") # Danger-based descriptions if danger > 7: descriptions.append("There's a palpable sense of threat in the air.") elif danger > 4: descriptions.append("You remain vigilant, aware that danger could lurk nearby.") else: descriptions.append("The area seems relatively safe, at least for now.") # Magic-based descriptions if magic > 7: descriptions.append("The air tingles with magical energy.") elif magic > 4: descriptions.append("Occasional flickers of arcane power can be sensed.") return " ".join(descriptions) # Example usage if __name__ == "__main__": ai = SimpleAI() print("Combat example:", ai.generate_combat_narrative("troll")) print("\nNPC example:", ai.generate_npc_interaction("merchant")) print("\nWeather example:", ai.generate_weather_description()) # Test responses test_inputs = [ "I want to attack the enemy", "I'll talk to the villager", "I look around carefully", "I continue on my journey" ] print("\nResponse examples:") for input_text in test_inputs: print(f"\nInput: {input_text}") print(f"Response: {ai.respond_to_input(input_text)}")
This simple AI system provides:
- Contextual responses based on player input
- A world state that evolves based on player actions
- Varied descriptions for combat, NPC interactions, and environments
- Time progression and weather effects
Step 5: Connecting to a Generative AI API (OpenAI)
Now, let's enhance our game with actual generative AI capabilities by connecting to the OpenAI API. First, we'll create a file for the AI integration:
# ai_integration.py import requests import json import os import random class GenerativeAI: def __init__(self, use_mock=True): """Initialize the GenerativeAI class. Args: use_mock: If True, use mock responses instead of actual API calls (useful for development or when you don't have an API key) """ self.use_mock = use_mock self.api_key = os.environ.get("OPENAI_API_KEY", "") # For demonstration purposes, we'll include mock responses self.mock_responses = { "story_hook": [ "Your journey begins when an ancient prophecy names you as the realm's last hope.", "A mysterious letter arrives, bearing your family's long-forgotten seal and a desperate plea for help.", "The village elder reveals you carry the bloodline of legendary heroes, just as ancient enemies return.", "What began as a simple errand becomes something far greater when you witness a falling star with a message meant only for you." ], "location_description": [ "The forest canopy forms a cathedral of leaves above you. Shafts of golden light pierce through, illuminating dancing dust motes and revealing glimpses of wildlife that watches your passage with ancient, knowing eyes. The path ahead winds between moss-covered stones that might once have been part of a forgotten civilization.", "This cave system extends far beyond what any map shows. Crystals embedded in the walls pulse with soft blue light, responding to your presence. The air carries a metallic taste and whispers that seem just at the edge of comprehension. Water drips form complex harmonies in distant chambers.", "The mountain peak pierces the cloud layer, offering you a view of the world that few have ever witnessed. The thin air makes your head light, but also sharpens your senses. Ancient carvings on weather-worn stones suggest this place has been sacred since before recorded history.", "The castle's grandeur has faded with centuries of neglect, yet its bones remain imposing. Tapestries, now faded and torn, hint at a noble history. Your footsteps echo through halls where hundreds once gathered, and you can't shake the feeling of being watched by the portraits whose eyes seem to follow your movement." ], "character_dialogue": [ "\"The stars speak of your coming,\" the old wizard says, eyes reflecting wisdom accumulated across centuries. \"But they remain silent about whether you bring salvation or further ruin. Which shall it be, I wonder?\"", "The merchant adjusts her elaborate headwrap, studying you with eyes sharp from decades of assessing both goods and character. \"Information is my most valuable commodity, traveler. What price are you willing to pay for what I know?\"", "\"Stand down or stand dead,\" the knight commands, armor gleaming despite the obvious signs of recent battle. \"These lands suffer enough without another wandering troublemaker. Prove your worth or be on your way.\"", "The child tugs at your sleeve with urgent insistence. \"The adults won't listen,\" they whisper, glancing nervously over their shoulder. \"But I've seen what hides in the abandoned well. It knows your name.\"" ], "combat_narrative": [ "The goblin pack employs surprising tactics, using the terrain to separate you from advantageous positions. Their crude weapons belie a cunning approach to combat that has evolved over generations of survival against stronger foes. You recognize their pattern just in time to turn their strategy against them.", "The wolf's eyes hold an intelligence that goes beyond animal instinct. It circles methodically, testing your defenses with feints and withdrawals. When it finally attacks, it targets not you but the strap of your pack, suggesting this isn't its first encounter with armed travelers.", "The bandit leader signals her companions with subtle hand gestures you recognize from military training. Their coordinated approach suggests former soldiers fallen on desperate times rather than common thieves. This changes your assessment of both the threat and potential resolution.", "The troll's regenerative abilities mean conventional combat tactics will fail. You notice its hesitation near your torch, revealing a vulnerability to fire that isn't mentioned in common folklore. This battle will require adaptation and environmental awareness rather than brute force." ], "quest_objective": [ "You must recover the Celestial Fragments, scattered when the Star Temple was destroyed. Each piece resonates with a different element and must be purified in its corresponding shrine before reassembly. The completed artifact will reveal the truth behind the realm's fading magic.", "The ancient boundary stones marking the kingdom's borders have been systematically defaced, weakening the protective enchantments laid by the realm's founders. You must restore each marker with the appropriate runes, working against unknown saboteurs who continue their work in the shadows.", "A diplomatic crisis looms between the mountain clans and forest kingdoms, with both sides preparing for war over suspected treachery. You must identify the true culprit—a third party sowing discord—by collecting evidence from crime scenes while navigating increasingly hostile territory.", "The seasonal rains have failed for the third year, and unnatural drought threatens the region. Your investigation reveals a corrupted water elemental bound in the central lake's depths. Freeing it requires gathering purification components from increasingly dangerous environments affected by the spreading corruption." ] } def generate_text(self, prompt, category, max_tokens=150): """Generate text using the OpenAI API or mock responses. Args: prompt: The prompt to send to the API category: Category for mock responses max_tokens: Maximum tokens in the response Returns: Generated text string """ if self.use_mock: # Return a mock response based on the category if category in self.mock_responses: return random.choice(self.mock_responses[category]) else: return "The AI ponders your request and provides a thoughtful response." # If not using mock responses, make an actual API call try: headers = { "Content-Type": "application/json", "Authorization": f"Bearer {self.api_key}" } data = { "model": "gpt-3.5-turbo", "messages": [{"role": "user", "content": prompt}], "max_tokens": max_tokens, "temperature": 0.7 } response = requests.post( "https://api.openai.com/v1/chat/completions", headers=headers, data=json.dumps(data) ) if response.status_code == 200: return response.json()["choices"][0]["message"]["content"].strip() else: print(f"API Error: {response.status_code}") print(response.text) # Fall back to mock responses on error if category in self.mock_responses: return random.choice(self.mock_responses[category]) return "The AI encountered an error but continues the story nonetheless." except Exception as e: print(f"Error calling OpenAI API: {str(e)}") # Fall back to mock responses on error if category in self.mock_responses: return random.choice(self.mock_responses[category]) return "The AI encountered an error but continues the story nonetheless." def generate_story_hook(self): """Generate a story hook for the game.""" prompt = "Create an intriguing story hook for a fantasy text adventure game. The hook should establish the player's initial quest or motivation." return self.generate_text(prompt, "story_hook") def generate_location_description(self, location_name): """Generate a rich description for a location.""" prompt = f"Describe a {location_name} in a fantasy world. Make the description vivid, atmospheric, and include sensory details." return self.generate_text(prompt, "location_description") def generate_character_dialogue(self, character_type): """Generate dialogue for an NPC character.""" prompt = f"Write a short, memorable dialogue line for a {character_type} in a fantasy setting. The dialogue should reveal something about their personality and hint at potential information or quests." return self.generate_text(prompt, "character_dialogue") def generate_combat_narrative(self, enemy_type): """Generate a combat narrative.""" prompt = f"Describe a tactical combat encounter with a {enemy_type} in a fantasy world. Focus on the enemy's fighting style and strategies rather than just physical description." return self.generate_text(prompt, "combat_narrative") def generate_quest_objective(self): """Generate a quest objective.""" prompt = "Create a detailed quest objective for a fantasy adventure that involves multiple steps and has environmental or political complexity beyond simple combat." return self.generate_text(prompt, "quest_objective") def generate_response_to_action(self, action, context): """Generate a response to a player's action.""" prompt = f"In a fantasy text adventure, the player is in {context} and performs this action: '{action}'. Describe the outcome in a detailed, atmospheric way." return self.generate_text(prompt, None, max_tokens=200) # Example usage if __name__ == "__main__": ai = GenerativeAI(use_mock=True) # Use mock responses print("Story Hook Example:") print(ai.generate_story_hook()) print("\nLocation Description Example:") print(ai.generate_location_description("ancient forest")) print("\nCharacter Dialogue Example:") print(ai.generate_character_dialogue("mysterious wizard")) print("\nCombat Narrative Example:") print(ai.generate_combat_narrative("goblin ambush")) print("\nQuest Objective Example:") print(ai.generate_quest_objective()) print("\nAction Response Example:") print(ai.generate_response_to_action("search the abandoned library for hidden passages", "a dusty castle library"))
This AI integration provides:
- Connection to the OpenAI API for generating text
- Mock responses for development or when an API key isn't available
- Specialized generation functions for different aspects of the game
- Error handling with fallback to mock responses
Step 6: Enhancing the Game with AI-Generated Content
Finally, let's create our ultimate version of the game that integrates all the components:
# ai_adventure_game.py import random import time import os from story_generator import StoryGenerator from simple_ai import SimpleAI from ai_integration import GenerativeAI class AITextAdventure: def __init__(self, player_name, use_advanced_ai=False): self.player_name = player_name self.health = 100 self.magic = 50 self.inventory = ["Map", "Torch", "Water Flask"] self.current_location = "Starting Point" self.game_active = True self.turn_count = 0 self.story_progress = 0 # Game elements self.locations = ["Forest", "Cave", "Mountain", "Castle", "Village", "Ruins", "Swamp", "Desert"] self.characters = ["Wizard", "Merchant", "Knight", "Mysterious Stranger", "Elder", "Child", "Guard", "Alchemist"] self.items = ["Sword", "Shield", "Potion", "Ancient Book", "Magic Amulet", "Crystal", "Key", "Enchanted Bow"] # Initialize AI systems self.story_gen = StoryGenerator() self.simple_ai = SimpleAI() self.gen_ai = GenerativeAI(use_mock=not use_advanced_ai) # Generate main quest if use_advanced_ai: self.main_quest = self.gen_ai.generate_story_hook() self.quest_objective = self.gen_ai.generate_quest_objective() else: self.main_quest = self.story_gen.generate_story_hook() self.quest_objective = "You must recover the lost artifacts and restore balance to the realm." self.plot_twist_revealed = False self.plot_twist = self.story_gen.generate_plot_twist() def display_status(self): """Show the player's current status.""" print(f"\n--- {self.player_name}'s Adventure ---") print(f"Location: {self.current_location}") print(f"Health: {self.health}") print(f"Magic: {self.magic}") print(f"Inventory: {', '.join(self.inventory)}") print(f"Quest: {self.main_quest}") print(f"Objective: {self.quest_objective}") def generate_location_description(self, location_name): """Generate a description for a location using the appropriate AI system.""" if random.random() < 0.7: # 70% chance to use advanced AI if available try: return self.gen_ai.generate_location_description(location_name) except: return self.story_gen.generate_location_description(location_name) else: return self.story_gen.generate_location_description(location_name) def generate_encounter(self): """Generate a random encounter.""" encounter_types = ["character", "item", "enemy", "puzzle", "rest"] encounter_type = random.choice(encounter_types) if encounter_type == "character": character = random.choice(self.characters) try: return self.gen_ai.generate_character_dialogue(character) except: return self.simple_ai.generate_npc_interaction(character) elif encounter_type == "item": item = random.choice(self.items) if item not in self.inventory: self.inventory.append(item) return f"You found a {item}! It has been added to your inventory." else: return f"You spot a {item}, but you already have one." elif encounter_type == "enemy": enemies = ["Goblin", "Wolf", "Bandit", "Skeleton", "Troll", "Dark Mage", "Giant Spider"] enemy = random.choice(enemies) damage = random.randint(5, 20) self.health -= damage try: narrative = self.gen_ai.generate_combat_narrative(enemy) return f"{narrative} You take {damage} damage in the fight." except: return self.simple_ai.generate_combat_narrative(enemy) + f" You take {damage} damage." elif encounter_type == "puzzle": return self.story_gen.generate_challenge() else: # rest health_gain = random.randint(5, 15) magic_gain = random.randint(5, 15) self.health = min(100, self.health + health_gain) self.magic = min(100, self.magic + magic_gain) rest_narratives = [ f"You find a peaceful clearing and take time to rest. You recover {health_gain} health and {magic_gain} magic.", f"A friendly hermit offers you shelter for a few hours. You recover {health_gain} health and {magic_gain} magic.", f"You discover a healing spring and take time to recuperate. You recover {health_gain} health and {magic_gain} magic." ] return random.choice(rest_narratives) def move_to_new_location(self): """Move to a new location.""" available_locations = [loc for loc in self.locations if loc != self.current_location] new_location = random.choice(available_locations) self.current_location = new_location return self.generate_location_description(new_location) def get_player_choice(self): """Get the player's next action choice.""" print("\nWhat would you like to do?") print("1. Explore current location") print("2. Move to a new location") print("3. Check status") print("4. Custom action (experimental)") print("5. Quit game") while True: try: choice = int(input("\nEnter your choice (1-5): ")) if 1 <= choice <= 5: return choice else: print("Please enter a number between 1 and 5.") except ValueError: print("Please enter a valid number.") def handle_custom_action(self): """Handle a custom player action using AI.""" action = input("\nWhat would you like to do? Describe your action: ") try: # Try to use the generative AI for a response context = f"the {self.current_location}" return self.gen_ai.generate_response_to_action(action, context) except: # Fall back to simple AI return self.simple_ai.respond_to_input(action) def advance_story(self): """Advance the main story based on turn count.""" self.turn_count += 1 self.story_progress = min(100, self.story_progress + random.randint(5, 10)) # Reveal plot twist around the middle of the game if self.story_progress >= 50 and not self.plot_twist_revealed: print("\n--- PLOT TWIST ---") print(self.plot_twist) self.plot_twist_revealed = True return True # Random story events if random.random() < 0.2: # 20% chance each turn story_events = [ "You find a torn page from an ancient book that hints at your destiny.", "A dream visits you in the night, showing glimpses of what's to come.", "Locals whisper about recent strange events that seem connected to your quest.", "You notice symbols that keep appearing, suggesting a pattern to your journey." ] print("\n--- STORY EVENT ---") print(random.choice(story_events)) return True return False def play_turn(self): """Play a single turn of the game.""" choice = self.get_player_choice() if choice == 1: # Explore print("\n" + self.generate_encounter()) elif choice == 2: # Move print("\n" + self.move_to_new_location()) elif choice == 3: # Check status self.display_status() elif choice == 4: # Custom action print("\n" + self.handle_custom_action()) elif choice == 5: # Quit self.game_active = False print("\nThanks for playing!") return # Advance story self.advance_story() # Check if player has lost if self.health <= 0: print("\nYour health has dropped to zero. Game over!") self.game_active = False # Check if story is complete if self.story_progress >= 100: print("\n--- QUEST COMPLETE ---") print("You have completed your adventure successfully!") print(f"Final status: Health {self.health}, Magic {self.magic}") print(f"Items collected: {', '.join(self.inventory)}") self.game_active = False def play_game(self): """Main game loop.""" print(f"\nWelcome, {self.player_name}, to your AI-powered text adventure!") print("\n--- YOUR QUEST ---") print(self.main_quest) print("\n--- YOUR OBJECTIVE ---") print(self.quest_objective) print("\n--- STARTING LOCATION ---") print(self.generate_location_description(self.current_location)) while self.game_active: self.play_turn() time.sleep(1) # Small pause between turns # Run the game if this file is executed directly if __name__ == "__main__": print("=== AI-POWERED TEXT ADVENTURE GENERATOR ===") print("This game uses AI to create a unique adventure experience.") player_name = input("Enter your character's name: ") use_advanced_ai = False api_key = os.environ.get("OPENAI_API_KEY") if api_key: use_advanced_ai = input("OpenAI API key detected. Use advanced AI generation? (y/n): ").lower() == 'y' else: print("No OpenAI API key found in environment variables.") print("The game will use simulated AI responses.") print("To use real AI, set the OPENAI_API_KEY environment variable.") game = AITextAdventure(player_name, use_advanced_ai) game.play_game()
This final version combines all our components:
- The basic game structure
- The story generator for varied narrative elements
- The simple AI for rule-based responses
- The generative AI integration for advanced content
- A custom action feature that uses AI to respond to free-form player input
Leveling Up - Next Steps in Python for AI
Now that you've built a text adventure game with AI elements, let's explore how you can take your Python skills further, especially for AI applications.
Advanced Python Concepts
As you continue your Python journey, consider exploring these more advanced concepts:
-
Object-Oriented Programming (OOP): We used classes in our game, but there's much more to learn about inheritance, polymorphism, and design patterns.
-
Decorators and Context Managers: These powerful Python features can make your code more elegant and efficient.
-
Asynchronous Programming: Using
async
andawait
for handling operations that might take time, like API calls. -
Type Hints: Adding type annotations to your code for better documentation and error catching.
-
Testing Frameworks: Learn about
pytest
orunittest
for proper testing of your code.
Key Python Libraries for AI
To go further with AI development, familiarize yourself with these essential libraries:
-
NumPy: The foundation of scientific computing in Python, providing efficient array operations.
-
Pandas: For data manipulation and analysis.
-
Matplotlib and Seaborn: For data visualization.
-
Scikit-learn: For machine learning algorithms.
-
TensorFlow or PyTorch: For deep learning.
Extending Your Text Adventure Project
Here are some ideas for extending your text adventure game:
-
Web Interface: Use Flask or Django to create a web interface for your game.
-
Voice Integration: Add text-to-speech and speech-to-text capabilities.
-
Persistent World: Save game state to a database so players can continue their adventures later.
-
Multiplayer Features: Allow multiple players to interact in the same world.
-
Image Generation: Integrate with image generation APIs to create visuals for locations and characters.
-
Advanced NLP: Implement more sophisticated natural language processing for understanding player commands.
-
Procedural Content Generation: Create algorithms to generate entire worlds, quests, and storylines.
Conclusion
You've now built a text adventure game that incorporates Python fundamentals and generative AI concepts. Along the way, you've learned about:
- Python syntax, data types, and control structures
- Functions and error handling
- Object-oriented programming with classes
- Working with external APIs
- Implementing AI capabilities in a practical project
This project serves as a foundation that you can continue to build upon as you explore more advanced Python concepts and AI technologies. The skills you've developed are transferable to many other types of applications, from web development to data science to more sophisticated AI systems.
Remember that learning programming is a journey, not a destination. Keep experimenting, building, and expanding your knowledge. The Python ecosystem is vast and constantly evolving, especially in the AI space, so there's always something new to discover.
Happy coding, and may your text adventures be ever engaging!
About the Author
This guide was created by an AI enthusiast with a passion for teaching programming concepts through practical, engaging projects. With experience in both Python development and generative AI applications, the author aims to make learning technical concepts fun and accessible to beginners.
Additional Resources
Python Learning Resources
- Official Python Documentation
- Real Python - Tutorials and articles
- Python for Everybody - Free course by Dr. Charles Severance
Generative AI Resources
- Hugging Face - Hub for AI models
- OpenAI Documentation
- Microsoft's Generative AI for Beginners
Community Forums
Books
- "Automate the Boring Stuff with Python" by Al Sweigart
- "Python Crash Course" by Eric Matthes
- "Generative Deep Learning" by David Foster
Tags
About the Author
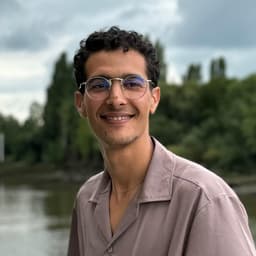
Ahmed Oublihi
A passionate Software Developer and technologist exploring the intersections of code and creativity.